Yahoo! JAPAN Ads Script | Developer Center
日本語Automatic upload of audience lists (customer data)
Script overview
Display Ads let advertisers upload a file of their customer data and import it into their audience lists.Yahoo! JAPAN Ads Script lets you automate this import process. By using automatic uploads, you can always use the latest data.
Create import files
See this help page for how to create import files.
Pattern 1: Automatically upload from Google Drive
Pattern 2: Upload from Google Sheets
Before you begin
For Patterns 1 and 2
1. Link your Yahoo! JAPAN Ads Script with your Google account.*If you have already linked your accounts, proceed to the next step.
2. Check the site retargeting ID in the Campaign Management Tool and make a note of it.
*For more detailed instructions, see this help page.
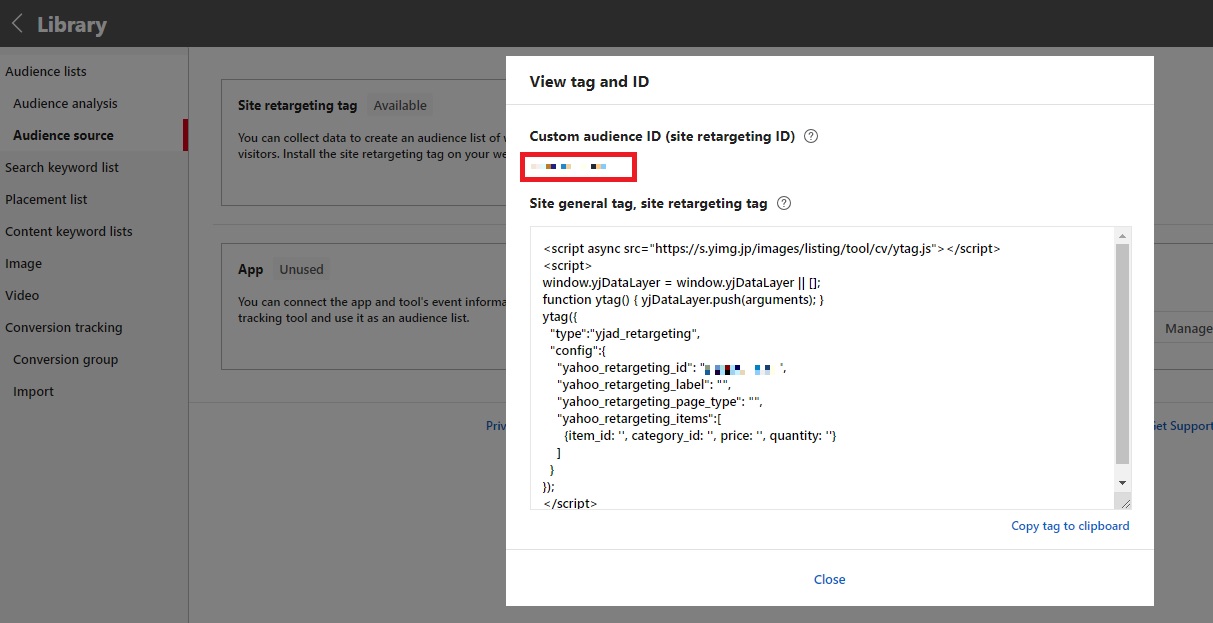
3. Check the audience list ID in the Campaign Management Tool and make a note of it.
*For more detailed instructions, see this help page.

For Pattern 1: When uploading from Google Drive
1. Upload a file for importing the audience list (customer data) to Google Drive.For file specifications, see this help page.
2. Get the file ID of the file you uploaded.
For Pattern 2: When uploading from Google Sheets
1. Enter the customer data in column A of the Google Sheet as shown in the following image (the example is aaid):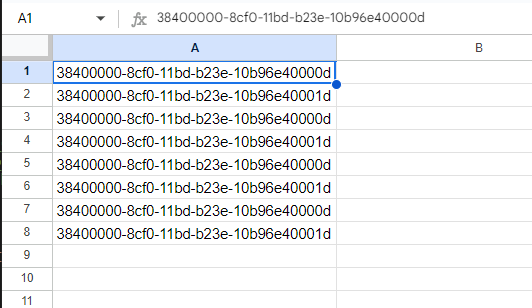
2. Get the spreadsheet ID.
Sample codes
Copy the following scripts, including comments in gray, and paste them into the script input, then set up the constants according to the instructions in the script.For Pattern 1: When uploading from Google Drive
/*
■Script summary
Upload your audience list (customer data) from the specified Google Drive file.
■How to use
1. Use this script as your Display Ads script.
2. Specify the constants as follows:
■Constants
・FLAG_MAIL: true if you want to email the results, false if you don't
・MAIL_TO: Yahoo! JAPAN Business ID of the email recipient
・MAIL_TITLE: Email title
・RETARGETING_TAGID: Check and specify the site retargeting ID in the Campaign Management Tool
・AUDIENCE_LISTID: Check and specify the audience list ID in the Campaign Management Tool
・FILE_ID: Specify the file ID on Google Drive
・UPLOAD_TYPE: Three types are available: IDFA, AAID, and MAIL_ADDRESS. For details, see this page→ https://ads-developers.yahoo.co.jp/en/ads-script/product-guide/reference/enums/display.AudienceListServiceUploadUserListUploadType.html
・COMPRESS_TYPE: "NONE" if no compression, "ZIP" if compressed in ZIP format
*/
const FLAG_MAIL = true;
const MAIL_TO = ['Yahoo! BusinessID'];
let MAIL_TITLE = 'Yahoo! JAPAN Ads Script: Automatic audience list upload';
const RETARGETING_TAGID = '1A2B3C4D5E';
const AUDIENCE_LISTID = 1234567890;
const FILE_ID = '11111AAAAAbbbbb_-222222BBBBccccc';
const UPLOAD_TYPE = 'AAID';
const COMPRESS_TYPE = 'NONE';
function main() {
const accountId = AdsUtilities.getCurrentAccountId();
try {
const fileData = DriveApp.getFileById(FILE_ID).getBlob().getBytes();
let uploadList = Display.AudienceListService.uploadUserList(accountId, RETARGETING_TAGID, AUDIENCE_LISTID, UPLOAD_TYPE, COMPRESS_TYPE, fileData).rval;
if (uploadList.values[0].operationSucceeded) {
sendEmail('Audience list automatic upload completed.');
} else {
throw new Error('An error occurred while uploading');
}
} catch (error) {
MAIL_TITLE = '!!Error!!' + MAIL_TITLE;
sendEmail('An error occurred during automatic upload of the audience list! Check the log in the Campaign Management Tool for details.\n' + error);
}
}
function sendEmail(text) {
if (FLAG_MAIL) {
MailApp.sendEmail({
to: MAIL_TO,
subject: MAIL_TITLE,
body: text,
});
} else {
// If no email is sent, output the log
Logger.log(text);
}
}
For Pattern 2: When uploading from Google Sheets
/*
■Script summary
Upload your audience list (customer data) from the specified Google Sheets.
■How to use
1. Use this script as your Display Ad.
2. Specify the constants as follows:
■Constants
・FLAG_MAIL: true if you want to email the results, false if you don't
・MAIL_TO: Yahoo! JAPAN Business ID of the email recipient
・MAIL_TITLE: Email title
・RETARGETING_TAGID: Check and specify the site retargeting ID in the Campaign Management Tool
・AUDIENCE_LISTID: Check and specify the audience list ID in the Campaign Management Tool
・SPREAD_SHEET_ID //Specify the spreadsheet ID (Reference: https://ads-developers.yahoo.co.jp/ja/ads-script/post/30390362.html#ssid)
・SHEET_NAME // Sheet name of the spreadsheet
・UPLOAD_TYPE: Three types are available: IDFA, AAID, and MAIL_ADDRESS. For details, please see here → https://ads-developers.yahoo.co.jp/ja/ads-script/product-guide/reference/enums/display.AudienceListServiceUploadUserListUploadType.html
・COMPRESS_TYPE: "NONE" if no compression, "ZIP" if compressed in ZIP format
*/
const FLAG_MAIL = true;
const MAIL_TO = ['Yahoo! BusinessID'];
let MAIL_TITLE = 'Yahoo! JAPAN Ads Script: Automatic audience list upload';
const RETARGETING_TAGID = '1A2B3C4D5E';
const AUDIENCE_LISTID = 1234567890;
const SPREAD_SHEET_ID = 'Spreadsheet ID';
const SPREAD_SHEET_NAME = 'Sheet 1'
const UPLOAD_TYPE = 'AAID';
const COMPRESS_TYPE = 'NONE';
function main() {
const accountId = AdsUtilities.getCurrentAccountId();
try {
let sh = SpreadsheetApp.openById(SPREAD_SHEET_ID).getSheetByName(SPREAD_SHEET_NAME);
let ssDataArray = sh.getDataRange().getValues();
let fileContent = '';
ssDataArray.forEach(row => {
fileContent += row[0] + '\n';
});
let blob = Utilities.newBlob(Buffer.from(fileContent, 'utf-8'));
let uploadList = Display.AudienceListService.uploadUserList(accountId, RETARGETING_TAGID, AUDIENCE_LISTID, UPLOAD_TYPE, COMPRESS_TYPE, blob).rval;
if (uploadList.values[0].operationSucceeded) {
sendEmail('Audience list automatic upload completed.');
} else {
throw new Error('An error occurred while uploading');
}
} catch (error) {
MAIL_TITLE = '!!Error!!' + MAIL_TITLE;
sendEmail('An error occurred during automatic upload of the audience list! Please check the log in the admin panel for details.\n' + error);
}
}
function sendEmail(text) {
if (FLAG_MAIL) {
MailApp.sendEmail({
to: MAIL_TO,
subject: MAIL_TITLE,
body: text,
});
} else {
// If no email is sent, output the log
Logger.log(text);
}
}
Check the results
Check the audience in the Campaign Management Tool. When the number of uploads appears in "User size," the process is complete.Tips
After you create an audience list, it may take up to 48 hours before the user size can be confirmed.
